Custom button Sign in Facebook swift 4
Facebook Login in Swift 4
First you must install CocoaPods facebook. To use the SDK with CocoaPods, add the following lines to your Podfile:
https://developers.facebook.com/docs/swift/getting-started
pod 'FacebookCore'
pod 'FacebookLogin'
pod 'FacebookShare' // if needed
Click this link to ragister your app for login https://developers.facebook.com/docs/facebook-login/ios. This is how costum button using .xib
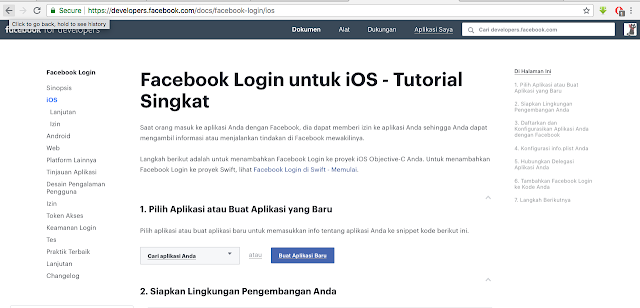
-- info.plist --> rightClick --> open As --> Source Code
Copy & Paste an XML snippet into your file contents (<dict> ... </ dict>).
<key>CFBundleURLTypes</key> <array> <dict> <key>CFBundleURLSchemes</key> <array> <string>fb(YourAppID)</string> </array> </dict> </array> <key>FacebookAppID</key> <string>(YourID)</string> <key>FacebookDisplayName</key> <string>(YourApp)</string>
- newfile --> cocoa touch class --> viewController
import UIKitimport FacebookLoginimport FBSDKLoginKitclass FacebookLoginViewController: UIViewController {@IBAction func bLoginF(_ sender: Any) {let loginManager = LoginManager()loginManager.logIn(readPermissions: [.publicProfile], viewController : self) { loginResult inswitch loginResult {case .failed(let error):print(error)case .cancelled:print("User cancelled login.")case .success(let grantedPermissions, let declinedPermissions, let accessToken):self.getFBUserData()}}}var dict : [String : AnyObject]!override func viewDidLoad() {super.viewDidLoad()//if the user is already logged inif (FBSDKAccessToken.current()) != nil{getFBUserData()}}//function is fetching the user datafunc getFBUserData() {if((FBSDKAccessToken.current()) != nil){FBSDKGraphRequest(graphPath: "me", parameters: ["fields": "id, name, picture.type(large), email"]).start(completionHandler: { (connection, result, error) -> Void inif (error == nil){self.dict = result as! [String : AnyObject]print(result!)print(self.dict)}})}}}
-- in your appdelegate.swift
1 . add func FBSDKapplicationDelegate -- > didFinishLaunchingWithOptions
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {FBSDKApplicationDelegate.sharedInstance().application(application, didFinishLaunchingWithOptions: launchOptions)window = UIWindow(frame: UIScreen.main.bounds)window?.makeKeyAndVisible()window?.rootViewController = UINavigationController(rootViewController: FacebookLoginViewController())return true}
2 . add func FBSDKAppEvents
func applicationWillResignActive(_ application: UIApplication) {
FBSDKAppEvents.activateApp()}
3 . add func FBSDKapplicationDelegate --> sourceApplication
func application(_ application: UIApplication, open url: URL, sourceApplication: String?, annotation: Any) -> Bool {
return FBSDKApplicationDelegate.sharedInstance().application(application, open: url, sourceApplication: sourceApplication, annotation: annotation)
}
full class AppDelegate
import UIKit
import FBSDKLoginKit
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
FBSDKApplicationDelegate.sharedInstance().application(application, didFinishLaunchingWithOptions: launchOptions)
window = UIWindow(frame: UIScreen.main.bounds)
window?.makeKeyAndVisible()
window?.rootViewController = UINavigationController(rootViewController: FacebookLoginViewController())
return true
}
func applicationWillResignActive(_ application: UIApplication) {
FBSDKAppEvents.activateApp()
}
func application(_ application: UIApplication, open url: URL, sourceApplication: String?, annotation: Any) -> Bool {
return FBSDKApplicationDelegate.sharedInstance().application(application, open: url, sourceApplication: sourceApplication, annotation: annotation)
}
func applicationDidEnterBackground(_ application: UIApplication) {
// Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
// If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
}
func applicationWillEnterForeground(_ application: UIApplication) {
// Called as part of the transition from the background to the active state; here you can undo many of the changes made on entering the background.
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
func applicationWillTerminate(_ application: UIApplication) {
// Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
}
}
No comments: